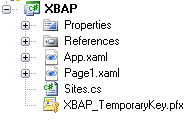
Open Page1.Xaml
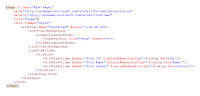
Place the above listview tags inside Grid .
Create a new class called Site.cs
using System;
using System.Collections.Generic;
namespace XBAP
{
public class Sites
{
public Sites(int _Id,string _Name,string _Url,string _Author)
{
this.Id = _Id;
this.Name = _Name;
this.Url = _Url;
this.Author = _Author;
}
public int Id {get;set;}
public string Name { get; set; }
public string Url { get; set; }
public string Author { get; set; }
}
}
Open page1.xaml.cs
Add the below code
public partial class Page1 : Page
{
public Page1()
{
InitializeComponent();
this.Loaded += new RoutedEventHandler(Page1_Loaded);
}
void Page1_Loaded(object sender, RoutedEventArgs e)
{
ListSites.ItemsSource = GetSites();
}
public List<Sites> GetSites()
{
List<Sites> lstSites = new List<Sites> ();
lstSites.Add(new Sites(1,"Yahoo Mail","http://mail.yahoo.com","YAHOO"));
lstSites.Add(new Sites(2, "AOL", "http://mail.aol.com", "AIM"));
lstSites.Add(new Sites(3, "HotMail", "http://mail.msn.com", "MSN"));
lstSites.Add(new Sites(4, "GMail", "http://mail.google.com", "Google"));
return lstSites;
}
}
Run It .